+ C#でXAML
created 2010-07-21 modified 2024-06-29
C#でXAMLでWPFなキューブが回るプログラムです。
少し前まで、3DプログラミングとGUIツールキットがうまく組み合わさっているものって知らなかった。ほとんど無かったと思います。ですが、これ(WPF)は、いいです。
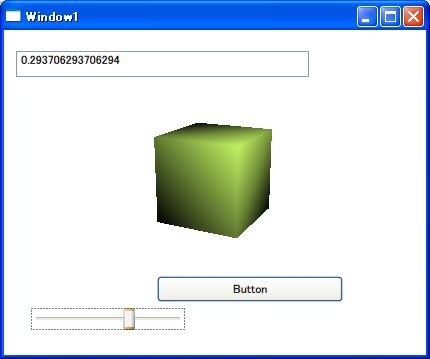
何かのお役に立てるなら、「修正済みBSDライセンス」または「X11ライセンス」でご利用ください。
少し前まで、3DプログラミングとGUIツールキットがうまく組み合わさっているものって知らなかった。ほとんど無かったと思います。ですが、これ(WPF)は、いいです。
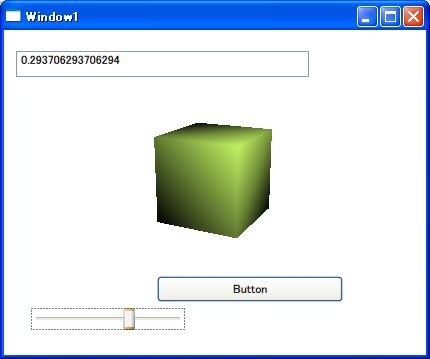
ダウンロードはこちら
ファイル | 備考 |
---|---|
WpfApplication1.zip | ソース |
WpfApplication1.exe | 実行ファイルのみ |
何かのお役に立てるなら、「修正済みBSDライセンス」または「X11ライセンス」でご利用ください。
修正済みBSDライセンスとは
詳しくは さまざまなライセンスとそれらについての解説 - GNU プロジェクト - フリーソフトウェア財団 (FSF) をどうぞ。Window1.xaml
<Window x:Class="WpfApplication1.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="Window1" Height="359" Width="430"> <Grid> <TextBox Name="textBox1" Margin="12,21,117,0" Height="26" VerticalAlignment="Top" /> <Button Name="button1" Click="button1_Click" Margin="153,0,83,53" Height="26" VerticalAlignment="Bottom" >Button</Button> <Slider Height="22" Margin="27,0,0,25" Name="slider1" VerticalAlignment="Bottom" HorizontalAlignment="Left" Width="154" ValueChanged="slider1_ValueChanged" Minimum="-1" Maximum="1" /> <Canvas Margin="27,66,12,97" Name="canvas1" > <Viewport3D Name="vp3d" ClipToBounds="True" Width="369" Height="158" Canvas.Left="0" Canvas.Top="0"> <Viewport3D.Camera> <PerspectiveCamera x:Name="camera" FieldOfView="45" NearPlaneDistance="0.1" FarPlaneDistance="50" Position="0,3,10" LookDirection="0,-3,-10" UpDirection="0,10,0"/> </Viewport3D.Camera> <ModelVisual3D x:Name="object3D"> <ModelVisual3D.Content> <GeometryModel3D> <GeometryModel3D.Geometry> <!-- 頂点集合を定義して、頂点のインデックスで三角の集合を定義する。 --> <!-- 三角を反時計回りに指定すれば、法線ベクトルは省略できるらしい。 --> <MeshGeometry3D Positions=" 1,1,1 -1,1,1 -1,-1,1 1,-1,1 1,1,-1 -1,1,-1 -1,-1,-1 1,-1,-1 " TriangleIndices=" 1,2,0 3,0,2 4,7,5 6,5,7 0,3,4 7,4,3 1,5,2 6,2,5 5,1,4 0,4,1 3,2,7 6,7,2 " Normals="" TextureCoordinates="" > </MeshGeometry3D> </GeometryModel3D.Geometry> <GeometryModel3D.Material> <MaterialGroup> <DiffuseMaterial> <DiffuseMaterial.Brush> <SolidColorBrush Color="#ccff66"/> </DiffuseMaterial.Brush> </DiffuseMaterial> </MaterialGroup> </GeometryModel3D.Material> </GeometryModel3D> </ModelVisual3D.Content> </ModelVisual3D> <ModelVisual3D x:Name="Light"> <ModelVisual3D.Content> <DirectionalLight Color="#FFFFFF" Direction="-5,-5,-5" /> </ModelVisual3D.Content> </ModelVisual3D> </Viewport3D> </Canvas> </Grid> </Window>
Window1.xaml.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; namespace WpfApplication1 { /// <summary> /// Window1.xaml の相互作用ロジック /// </summary> public partial class Window1 : Window { Viewport3D vp; PerspectiveCamera cam; const Double camR = 10.0; public Window1() { InitializeComponent(); vp = (Viewport3D)canvas1.Children[0]; cam = (PerspectiveCamera)vp.Camera; } private void button1_Click(object sender, RoutedEventArgs e) { DateTime dt = DateTime.Now; textBox1.Text = dt.ToString(); } private void slider1_ValueChanged(object sender, RoutedPropertyChangedEventArgs<double> e) { textBox1.Text = slider1.Value.ToString(); Double x, z; x = Math.Sin(slider1.Value * Math.PI / 2); z = Math.Cos(slider1.Value * Math.PI / 2); Point3D p; p = cam.Position; p.X = x * camR; p.Z = z * camR; cam.Position = p; Vector3D v; v = cam.LookDirection; v.X = -x * camR; v.Z = -z * camR; cam.LookDirection = v; } } }